备忘一下这个底部输入框的效果:
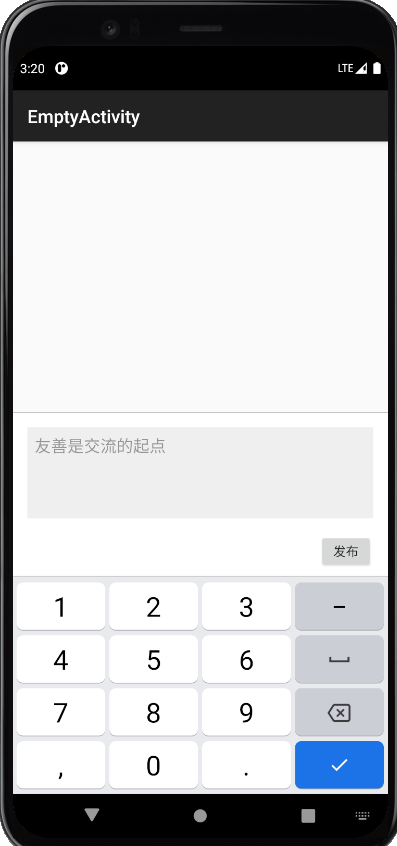
用DialogFragment实现挺方便的,大致的方法就是创建一个底部DialogFragment,布局内EditText聚焦,用代码主动弹出软键盘来。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| class BottomInputFragment : DialogFragment(){
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setStyle(STYLE_NO_TITLE, R.style.BottomInputDialog) } override fun onStart() { super.onStart() val window = dialog!!.window dialog!!.setCanceledOnTouchOutside(true) val params = window!!.attributes params.gravity = Gravity.BOTTOM params.width = WindowManager.LayoutParams.MATCH_PARENT window.attributes = params } override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? { return inflater.inflate(R.layout.dialog_edittext, container, false) }
override fun onViewCreated(view: View, savedInstanceState: Bundle?) { super.onViewCreated(view, savedInstanceState)
val editText = view.findViewById<EditText>(R.id.editText) showSoftInputFromWindow(editText) }
fun showSoftInputFromWindow(editText: EditText) { editText.isFocusable = true editText.isFocusableInTouchMode = true editText.requestFocus() val inputManager: InputMethodManager = editText.context.getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager inputManager.showSoftInput(editText, 0) } }
|
弹框的Style R.style.BottomInputDialog
1 2 3 4 5 6 7
| <style name="BottomInputDialog" parent="AppTheme"> <item name="android:layout_width">match_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:windowIsFloating">true</item> <item name="android:backgroundDimEnabled">false</item> <item name="android:windowSoftInputMode">stateAlwaysVisible</item> </style>
|
弹框的布局 R.layout.dialog_edittext
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:background="#fff" android:layout_width="match_parent" android:layout_height="wrap_content">
<View android:layout_width="match_parent" android:layout_height="1px" android:background="#3e3e3e"/>
<EditText android:id="@+id/editText" android:layout_width="match_parent" android:layout_height="100dp" android:padding="8dp" android:gravity="start|top" android:background="#efefef" android:layout_margin="16dp" android:maxLines="1" android:focusable="true" android:focusableInTouchMode="true" android:hint="友善是交流的起点" android:inputType="textMultiLine"/>
<Button android:layout_width="60dp" android:layout_height="40dp" android:layout_gravity="end" android:layout_marginEnd="16dp" android:layout_marginBottom="8dp" android:textSize="14sp" android:text="发布"/> <View android:layout_width="match_parent" android:layout_height="1px" android:background="#3e3e3e"/> </LinearLayout>
|
调用
1 2 3 4 5
| fun show() { val fm: FragmentManager = supportFragmentManager val bif = BottomInputFragment() bif.show(fm, "bif") }
|