请尽量使用:NotificationCompat 及其子类,以及 NotificationManagerCompat。
这样一来,您就无需编写条件代码来检查 API 级别,因为这些 API 会为您代劳。
但是要注意 NotificationManagerCompat 不能创建Android8.0以上所需要的Channel,创建Channel还是需要用NotificationManager。
简单的一个通知
1 2 3 4 5 6 7 8 9 10 11
| String channelId = "news"; NotificationCompat.Builder builder = new NotificationCompat.Builder(getApplicationContext(), channelId) .setSmallIcon(R.mipmap.ic_launcher) .setContentTitle("测试Title") .setContentText("一些简单的内容!!!");
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(getApplicationContext());
int notifyId = 123; notificationManager.notify(notifyId, builder.build());
|
但这个通知在Android8.0不能发出去,因为8.0规定必须将单个通知放入特定Channel中。
兼容Android8.0的通知
先 创建 NotificationChannel ,才能发出 Notification 。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| NotificationManager manager = (NotificationManager) context.getSystemService( Context.NOTIFICATION_SERVICE); String channelId = String.valueOf(notifyId);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { NotificationChannel channel = new NotificationChannel(channelId, "警报通知", NotificationManager.IMPORTANCE_HIGH); channel.enableLights(true); channel.setLightColor(ContextCompat.getColor(context, R.color.notifyChannel)); channel.setShowBadge(true); channel.setDescription("警报"); manager.createNotificationChannel(channel);
}
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(context, channelId); mBuilder.setContentTitle(title) .setContentText(msg) .setLargeIcon(BitmapFactory.decodeResource(context.getResources(), R.drawable.notification_image)) .setOnlyAlertOnce(true) .setDefaults(Notification.DEFAULT_ALL) .setWhen(System.currentTimeMillis()) .setSmallIcon(R.drawable.notification_icon) .setColor(ContextCompat.getColor(context, R.color.notifySmallIcon));
mBuilder.setAutoCancel(true);
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(context); notificationManager.notify(notifyId, mBuilder.build());
|
notification_icon

notification_image
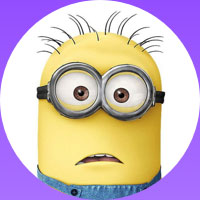
Genymotion模拟器显示效果
这里不测试国内的定制 ROM 效果,每个ROM都有自己的优化。
1. Android 4.4(API 级别 19 和 20)
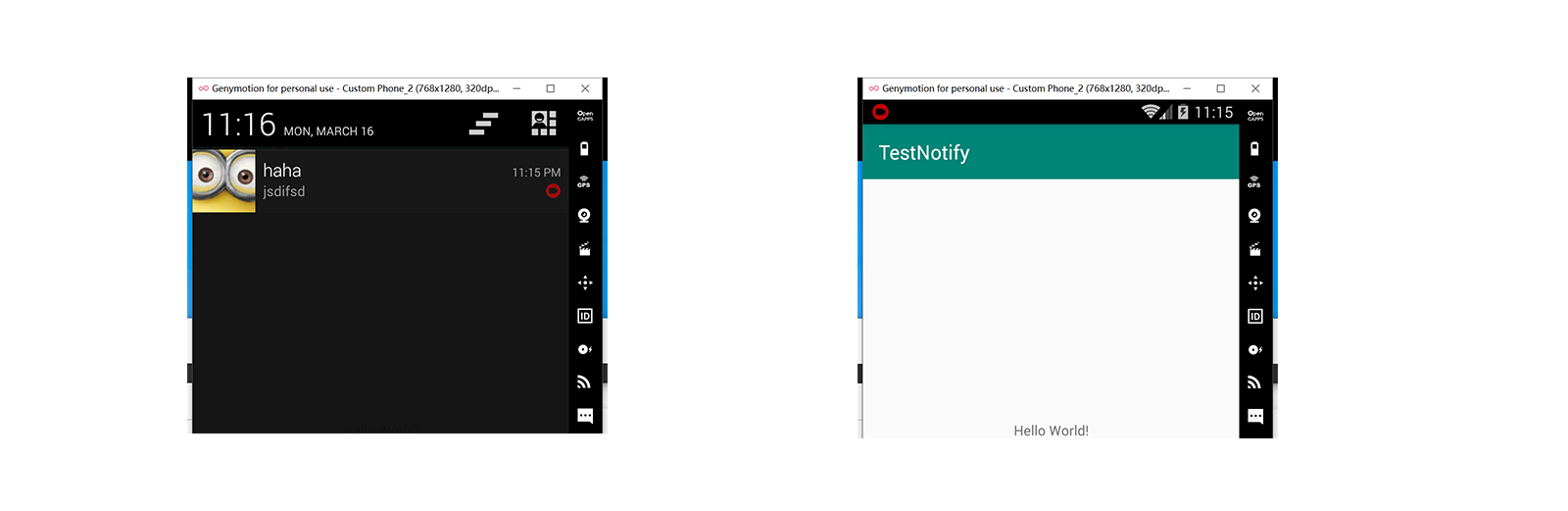
2. Android 5.0(API 级别 21)
- setSmallIcon的图标是镂空的,单色。
- 引入了锁定屏幕和浮动通知。
- 向 API 集添加了通知是否在锁定屏幕上显示的方法 (setVisibility()),以及指定通知文本的“公开”版本的方法。
单色Icon示例,白色部分是透明

2. Android 6.0(API 级别 23)
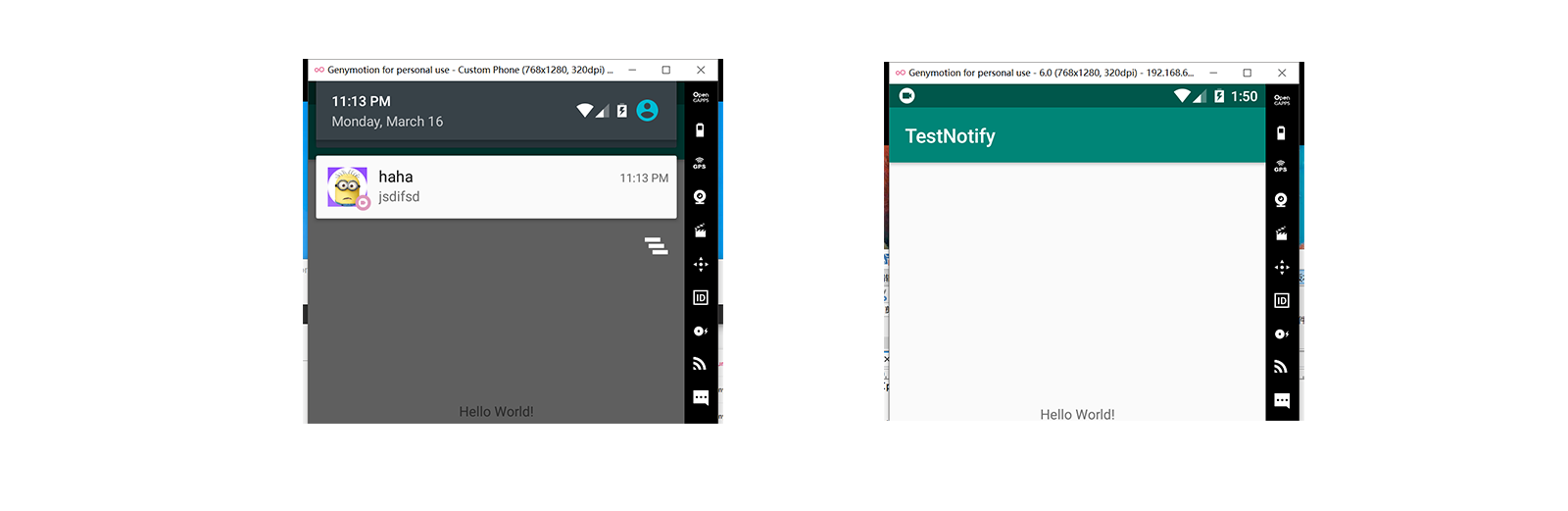
3. Android 7.0(API 级别 24)
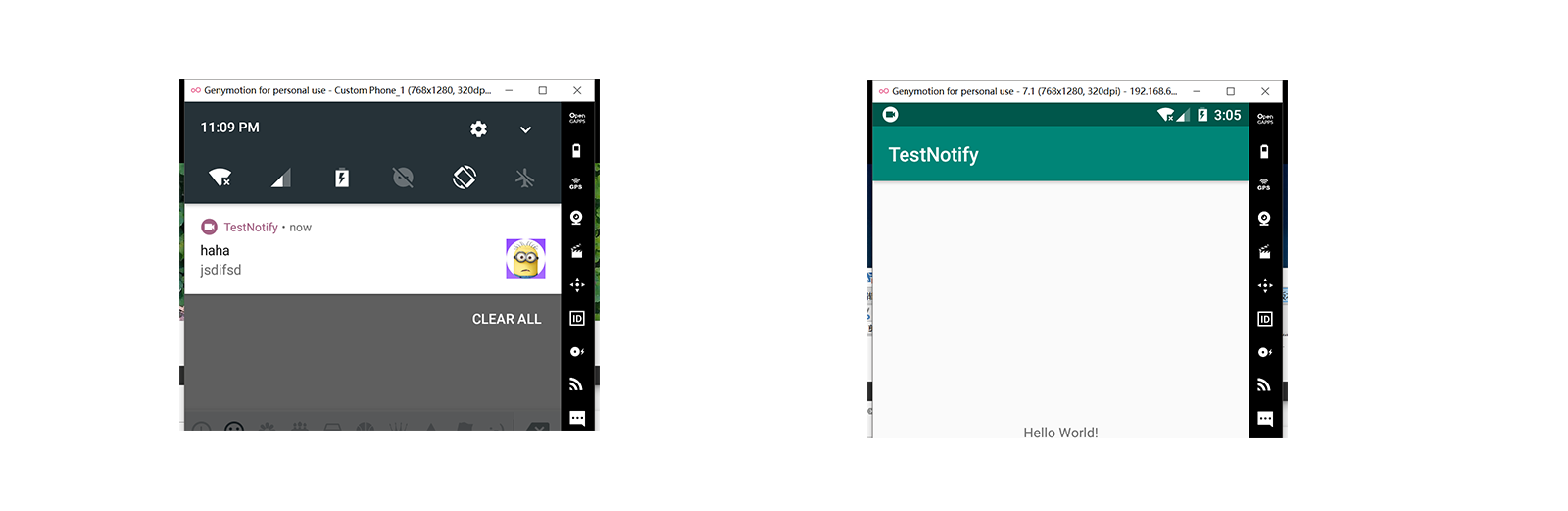
4. Android 8.0(API 级别 26)
- 现在必须将单个通知放入特定渠道中。
- 用户现在可以按渠道关闭通知,而不是关闭应用的所有通知。
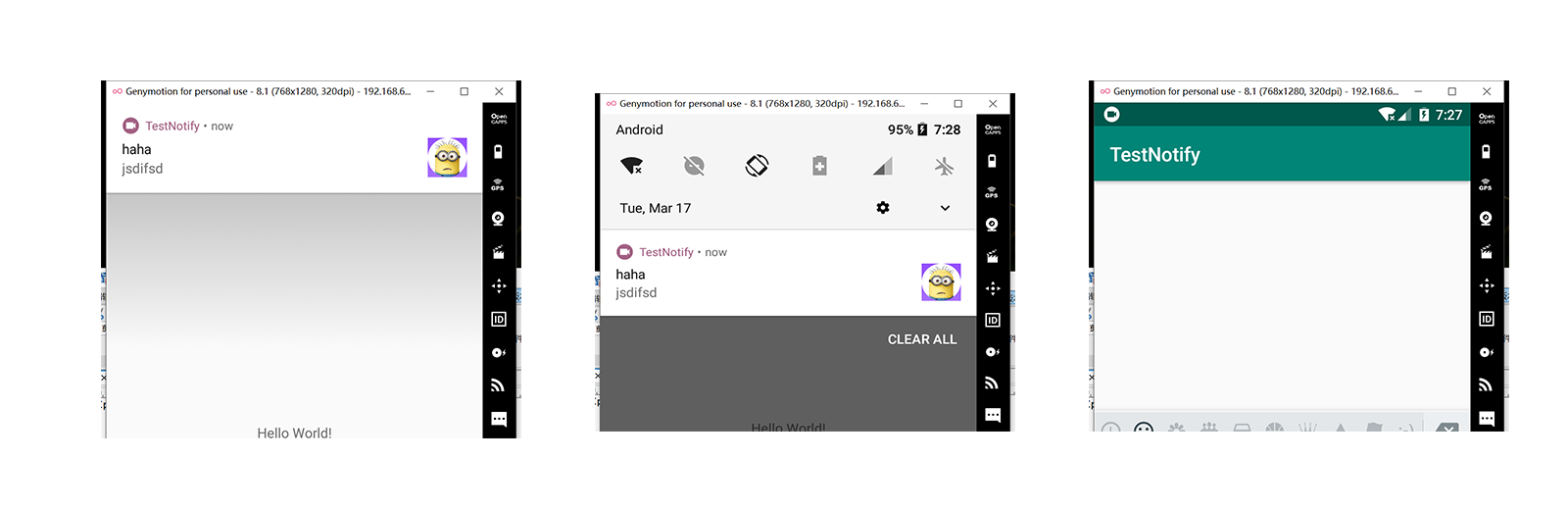
4. Android 9.0(API 级别 28)
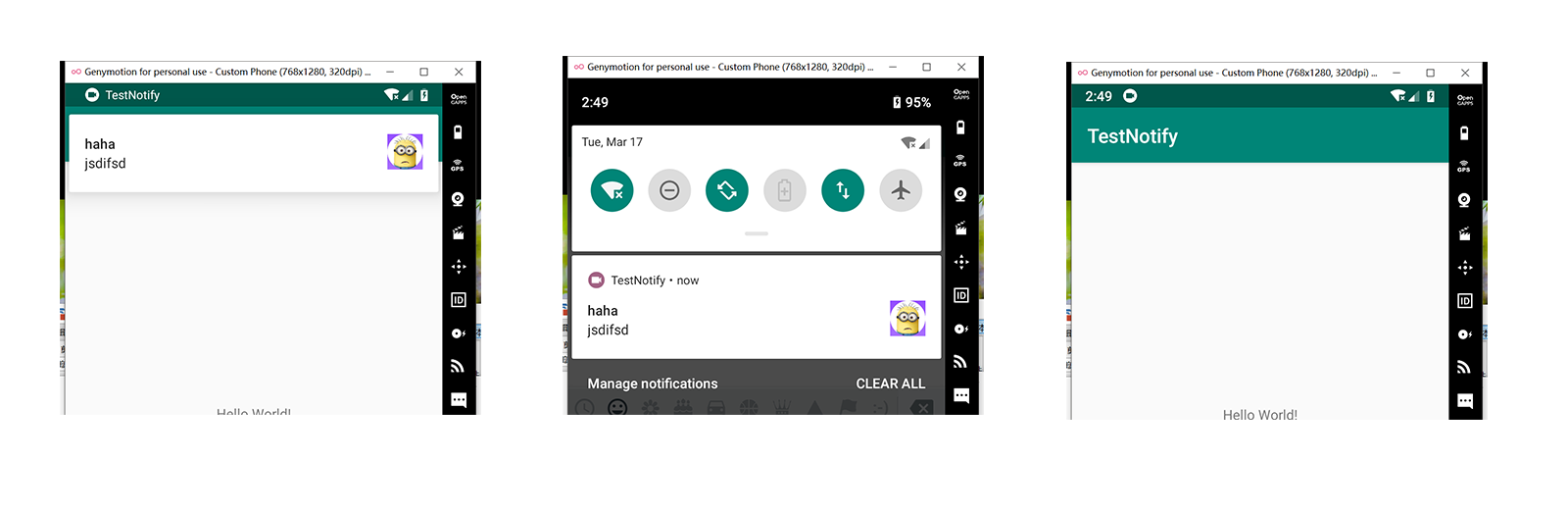
从通知启动 Activity
docs